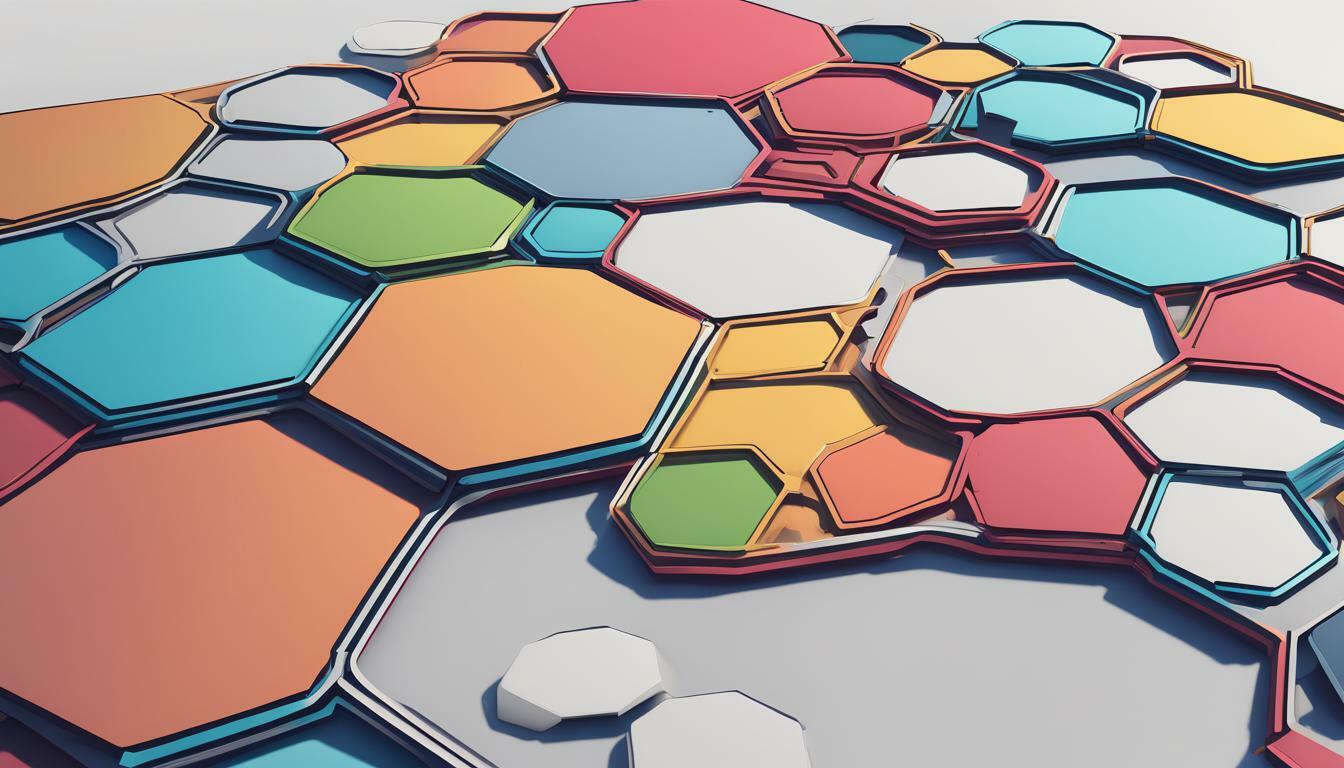
Welcome to our comprehensive guide to Hexagonal Architecture (Ports and Adapters) in software development. In this article, we’ll take a closer look at this powerful architectural pattern and how it can improve the modularity, testability, and maintainability of your projects.
Hexagonal Architecture, also known as Ports and Adapters, is a software design pattern that emphasizes the separation of concerns between the application core and its external dependencies. This pattern is gaining popularity due to its ability to create modular, flexible, and scalable software systems.
At its core, Hexagonal Architecture revolves around the concept of ports and adapters. Ports define the boundaries of the application core, while adapters connect the core to external systems, such as databases, user interfaces, and APIs.
In the following sections, we’ll provide an in-depth explanation of Hexagonal Architecture and how it works. We’ll also explore the benefits of this pattern, as well as provide concrete examples of its implementation.
Key Takeaways
- Hexagonal Architecture (Ports and Adapters) is a software design pattern that emphasizes the separation of concerns between the application core and its external dependencies.
- Ports and Adapters define the boundaries of the application core and connect it to external systems, respectively.
- Hexagonal Architecture improves modularity, testability, and maintainability in software development.
- Concrete examples of Hexagonal Architecture and Ports and Adapters will be provided in this article.
Understanding Hexagonal Architecture
Hexagonal Architecture, also known as Ports and Adapters, is a powerful software design pattern that emphasizes the separation of concerns in software systems. It is a paradigm that emphasizes modularity and testability by decoupling the application core from the input and output components.
At its core, Hexagonal Architecture is a means of dividing the application into different domains, each with a specific responsibility. By isolating these domains, it allows for the flexibility to change one area of the application without affecting another.
One of the key differences between Hexagonal Architecture and other architectural patterns is that it puts the application at the center. The application is seen as a hexagon with input and output ports connected to the outside world. The ports act as interfaces between the application and the external systems. The adapters, on the other hand, are responsible for translating the data received by the ports to a format that the application can understand.
By separating the application from the external systems, Hexagonal Architecture provides a way to keep the business logic independent of the delivery mechanism. This allows for flexibility in the choice of technologies used for the input and output ports. It also simplifies the testing of the application logic by allowing for the creation of isolated test environments.
The key to understanding Hexagonal Architecture is to focus on the idea of decoupling. By separating the core application logic from external dependencies, it allows for the creation of a more modular and flexible system. This makes it easier to maintain and upgrade the system in the long run.
The Ports and Adapters Pattern
The Ports and Adapters pattern is a key component of Hexagonal Architecture. It is designed to facilitate the decoupling of dependencies between different software layers. The pattern achieves this by providing a clear separation between the application core and the infrastructure that surrounds it.
At its core, the Ports and Adapters pattern consists of two main elements: ports and adapters. Ports are the interfaces through which the application core communicates with the external world. Adapters are the components that implement these interfaces, translating the requests and responses between the core and the outside world.
The Ports and Adapters pattern is particularly useful in complex software systems where there are multiple external dependencies. By separating the infrastructure from the application core, the pattern allows for greater flexibility and modularity. This, in turn, makes it easier to maintain and scale the system over time.
Hexagonal Architecture Explained
Hexagonal Architecture, also known as Ports and Adapters, is a software development pattern that emphasizes the decoupling of application logic from external dependencies. It achieves this by defining a clear separation between the core application logic and its inputs and outputs through a set of interfaces called ports and adapters.
The core of the application is responsible for implementing the domain-specific business rules and contains no external dependencies. The input and output ports serve as the means through which external systems can communicate with the application, while the adapters are responsible for bridging the gap between the application and the external dependencies.
The beauty of Hexagonal Architecture lies in its ability to enable developers to build software that is modular, testable, and easy to maintain. By decoupling the application logic from external dependencies, changes to either one can be made without affecting the other, making it easier to adapt the application to new requirements or integrate it with new technologies.
Overall, Hexagonal Architecture is a powerful approach to designing software that can help developers build robust, flexible, and maintainable applications.
Hexagonal Architecture Example
Let’s take a look at an example to better understand how Hexagonal Architecture can improve software development. Consider a simple e-commerce application that enables customers to purchase products online. The application has two main components: the front-end user interface and the back-end product inventory management system.
In a traditional layered architecture, the user interface would be tightly coupled to the inventory management system. Any change to the inventory management system would require modifications to the presentation layer as well. This can lead to a brittle and inflexible system that is difficult to maintain.
However, in a Hexagonal Architecture, these two components are decoupled by defining input and output ports between them. The input port in the UI layer allows the user to request a product, while the output port in the inventory management system layer returns the requested product data. By using this approach, the two components can evolve independently without affecting each other.
Let’s say the business expands and decides to add a new payment processing system. In a layered architecture, this change would require modifications to both the presentation layer and the inventory management system. However, in a Hexagonal Architecture, the payment processing system can be added as a new adapter, which implements the required ports. The existing system components remain unchanged, and the new system can be integrated without affecting the existing components.
By adopting this approach, Hexagonal Architecture ensures that software systems are modular and maintainable. The decoupling of components also leads to better testability, as each component can be tested independently.
Benefits of Hexagonal Architecture
Hexagonal Architecture, also known as Ports and Adapters, is becoming increasingly popular as a software development pattern due to its numerous benefits.
Modularity: By separating the application core from its external dependencies, Hexagonal Architecture allows for easy module replacement and integration. This enhances the modularity of the software, making it more flexible and scalable.
Testability: With Hexagonal Architecture, testing becomes much easier as the application core can be tested in isolation from its external dependencies. This eliminates the need for complex test setups and improves the reliability of the tests.
Maintainability: By isolating external dependencies, Hexagonal Architecture enhances the maintainability of the software. Changes to external systems can be made without affecting the core application logic, making maintenance tasks much easier.
Flexibility: Hexagonal Architecture allows for greater flexibility in software development. It enables the software to adapt to changing requirements and external dependencies.
Overall, the benefits of Hexagonal Architecture make it a valuable pattern for creating robust and flexible software systems.
Ports and Adapters Explained
One of the core elements of Hexagonal Architecture is the Ports and Adapters pattern. This pattern enables developers to decouple an application’s core logic from its external dependencies. But what exactly are ports and adapters, and how do they work?
Put simply, a port is an interface that defines a set of operations supported by the application. An adapter, on the other hand, is responsible for implementing these operations and providing access to external systems and resources.
Ports and adapters work together to enable communication between the application core and its external dependencies. By defining these interfaces, developers can ensure that their code is modular and can be easily tested and maintained.
For example, let’s assume that an application needs to retrieve data from a third-party API. Instead of directly making HTTP requests from the application core, developers can define a “data access” port. This port can then be implemented by an adapter that handles the HTTP requests and provides the response to the application core.
Ports
Ports are the interfaces that the application core uses to communicate with the outside world. These interfaces define the set of operations that the application needs to perform and the data that it requires to perform them. The core has no knowledge of the implementation behind the ports; it only knows how to call their operations.
Ports can be classified into two categories: input ports and output ports. Input ports represent the entry point to the application, while output ports are used to send data out of the application.
Adapters
Adapters are responsible for implementing the interfaces defined by the ports. They provide access to external systems and resources and transform data from one format to another as required by the application core.
There are two types of adapters that can be used with ports: inbound adapters and outbound adapters. Inbound adapters are used to transform incoming data into the format required by the application core. Outbound adapters, on the other hand, are used to transform data from the application into a format that can be used by external systems.
By using adapters, developers can ensure that the application core is completely decoupled from its external dependencies. This makes the code more modular and easier to test, maintain, and extend over time.
Ports and Adapters Example
Let’s take a look at a practical example of how the Ports and Adapters pattern can be used to integrate external services and components in a software project.
Suppose we are tasked with building a web application that allows users to search for flight bookings. In order to provide accurate and up-to-date flight information, we need to integrate with a third-party flight booking service. To do so, we can use the Ports and Adapters pattern in the following way:
Component | Description |
---|---|
Input Ports | These are the interfaces that the web application uses to interact with the application core. In this example, the input port would be the API endpoint that receives flight search requests from the user. |
Output Ports | These are the interfaces that the application core uses to interact with external systems. In this example, the output port would be the interface that communicates with the flight booking service API. |
Adapters | These are the concrete implementations of the input and output ports. They are responsible for translating between the domain model used by the application core and the data formats required by the external systems. In this example, we would need an adapter to communicate with the flight booking service API, as well as an adapter to format the response data into our application’s domain model. |
By using this pattern, we can achieve a high level of modularity and testability in our software project. We can easily swap out adapters to use different external services, or even mock out the adapters for testing purposes.
Overall, the Ports and Adapters pattern is a powerful tool for building flexible and maintainable software systems.
Benefits of Ports and Adapters
The Ports and Adapters pattern offers numerous benefits to software development. By separating the application core from external dependencies, it allows for modularity and flexibility in design. This, in turn, facilitates easy maintenance and scalability of the software system.
The use of input and output ports in the Ports and Adapters pattern enables straightforward testing and debugging of the application’s components. The decoupling of dependencies also allows for the integration of new components and external services without affecting the application core.
The Ports and Adapters pattern promotes code reusability and clean architecture by keeping the application core free of external system details. This makes the code more readable and maintainable, reducing the time and effort required for troubleshooting and updates.
The pattern’s use of adapters allows for the integration of legacy systems and third-party services without impacting the application’s functionality. This enables the development of applications that can easily adapt to changing business requirements and technology trends.
In summary, the Ports and Adapters pattern enhances the testability, maintainability, scalability, and flexibility of software systems. Understanding and incorporating it into software development can lead to more robust and efficient applications that can adapt to evolving business needs.
Combining Hexagonal Architecture and Ports and Adapters
While Hexagonal Architecture and Ports and Adapters are powerful design patterns on their own, combining them can lead to even better results. The Ports and Adapters pattern can be used to implement the input and output ports of the Hexagonal Architecture, and the adapters can be used to integrate external systems with the core application.
The Ports and Adapters pattern provides a clear separation between the application core and the outside world, allowing for easy testing and modularity. The Hexagonal Architecture provides a clear separation between the business logic and the infrastructure, enabling scalability and flexibility. Combining these two patterns allows for a modular and scalable architecture that is easy to maintain and test.
By using the Ports and Adapters pattern to implement the input and output ports, you can easily swap out different implementations without affecting the application core. This allows for more flexibility and easier maintenance. Adapters can be used to integrate external systems, such as databases or third-party APIs, with the application core. This makes it easier to handle changes to external systems without affecting the core logic of the application.
Combining these two patterns also allows for better error handling. The Ports and Adapters pattern provides clear boundaries between the application and external systems, making it easier to handle errors and exceptions. The Hexagonal Architecture allows for easy testing of the application logic, enabling developers to catch errors before they impact the application.
In conclusion, combining the Hexagonal Architecture and Ports and Adapters patterns can lead to a flexible, scalable, and maintainable software design. By using the Ports and Adapters pattern to implement the input and output ports, and the adapters to integrate external systems, you can create a modular architecture that is easy to test and maintain.
Conclusion
Hexagonal Architecture (Ports and Adapters) is a powerful pattern that offers numerous benefits for modern software development. By adopting Hexagonal Architecture, developers can improve the modularity, flexibility, and testability of their projects. By decoupling dependencies and enhancing maintainability, Hexagonal Architecture can help developers create more robust and scalable systems.
The Ports and Adapters pattern is the foundation of Hexagonal Architecture, providing a means of facilitating the integration of external systems while maintaining loose coupling. By incorporating Ports and Adapters into their designs, developers can create more flexible and robust architectures that are better equipped to handle changes and adapt to evolving user needs.
By combining Hexagonal Architecture and Ports and Adapters, developers can create truly powerful and flexible software designs that are capable of meeting the demands of modern development. Whether working on small projects or large-scale enterprise applications, Hexagonal Architecture (Ports and Adapters) is a valuable tool for any software developer.
Key Takeaways
- Hexagonal Architecture (Ports and Adapters) offers numerous benefits for modern software development
- The Ports and Adapters pattern is the foundation of Hexagonal Architecture and provides a means of facilitating the integration of external systems while maintaining loose coupling
- By combining Hexagonal Architecture and Ports and Adapters, developers can create truly powerful and flexible software designs that are capable of meeting the demands of modern development
- Understanding and applying Hexagonal Architecture (Ports and Adapters) is crucial for creating robust and scalable software systems
FAQ
Q: What is Hexagonal Architecture (Ports and Adapters)?
A: Hexagonal Architecture, also known as Ports and Adapters, is a software architectural pattern that promotes loose coupling and modularity. It separates the core business logic from external dependencies, such as databases, APIs, and user interfaces.
Q: How does Hexagonal Architecture differ from other architectural patterns?
A: Hexagonal Architecture focuses on the flow of data and interactions within the system, rather than the specific technologies used. It prioritizes testability, flexibility, and maintainability by decoupling the application from its external dependencies.
Q: What are the components of Hexagonal Architecture?
A: Hexagonal Architecture consists of several components, including the application core, input and output ports, and adapters. The application core contains the business logic, while the ports define the interfaces for interacting with external systems. Adapters provide the implementation details for these interactions.
Q: Can you provide an example of how Hexagonal Architecture is implemented?
A: Sure! Let’s say you have an e-commerce application. The application core would handle the business logic, such as processing orders and managing inventory. The input ports would define interfaces for receiving requests, and the output ports would define interfaces for interacting with external systems, such as a payment gateway or a shipping API. The adapters would provide the implementation details for these interactions, allowing the application to be easily tested and adapted to different environments.
Q: What are the benefits of using Hexagonal Architecture?
A: Hexagonal Architecture offers several benefits, including improved testability, modularity, and maintainability. It allows for easier integration of external systems and promotes flexible and scalable software design. With Hexagonal Architecture, changes to external dependencies can be easily managed without affecting the core business logic.
Q: What is the Ports and Adapters pattern?
A: The Ports and Adapters pattern, also known as Hexagonal Architecture, is a software design pattern that focuses on separating the application’s core logic from its external dependencies. It uses ports to define interfaces for interacting with external systems and adapters to provide the implementation details for these interactions.
Q: How does the Ports and Adapters pattern facilitate decoupling of dependencies?
A: The Ports and Adapters pattern achieves decoupling by defining clear interfaces between the application and its external dependencies, such as databases or APIs. These interfaces, or ports, provide a way for the application to interact with external systems without being tightly coupled to their specific implementation. Adapters then provide the necessary bridge between the application and the external systems.
Q: Can you provide an example of how the Ports and Adapters pattern is used?
A: Absolutely! Let’s take the example of a music streaming service. The core application logic would handle features like searching for songs, creating playlists, and playing music. The input ports would define interfaces for receiving user requests, while the output ports would define interfaces for interacting with external services like a music database or a payment gateway. Adapters would then provide the implementation details for these interactions, allowing the application to be easily tested and integrated with different external systems.
Q: What are the benefits of incorporating the Ports and Adapters pattern in software design?
A: The Ports and Adapters pattern offers several benefits, including improved modularity, testability, and maintainability. It allows for easier integration with external systems, promotes separation of concerns, and enables the application to be easily adapted to different environments or technologies.
Q: How can Hexagonal Architecture and Ports and Adapters be combined?
A: Hexagonal Architecture and Ports and Adapters are closely related concepts, as Hexagonal Architecture is sometimes referred to as the Ports and Adapters pattern. They can be combined by using the principles of Hexagonal Architecture to structure the overall system and applying the specific implementation details of the Ports and Adapters pattern for interacting with external systems.